Understanding Big O Notation: A Beginner’s Guide
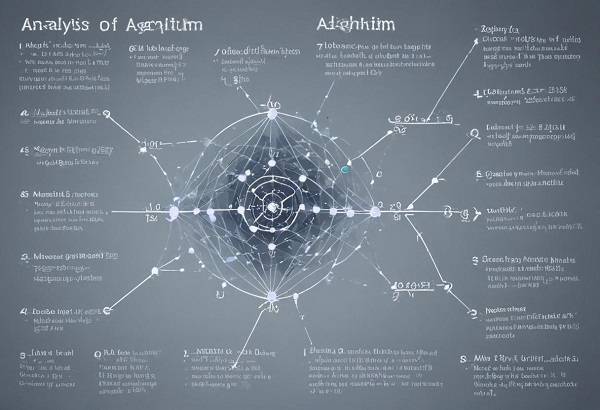
Introduction to Big O Notation
When analyzing the efficiency of algorithms, it’s crucial to understand how well they scale with larger input sizes. That’s where Big O Notation comes into play. Big O Notation provides a way to express an algorithm’s time complexity, allowing us to compare different algorithms based on how they perform as inputs grow. In this blog, we’ll explore Big O, its importance, and examples of common complexities.
Why Big O Notation?
Imagine you have two algorithms that perform the same task, but one is noticeably faster than the other for large inputs. Big O Notation helps us understand why. It gives us a mathematical way to analyze an algorithm’s performance by focusing on the most significant factors that impact execution time. Rather than focusing on exact execution time, Big O looks at how performance scales as inputs grow.
Key Terms to Know
- n: Input size
- Time Complexity: Measures how the time needed by an algorithm grows as the input size increases.
- Space Complexity: Measures how much memory an algorithm requires relative to the input size.
Big O Complexity Classes
Here’s a look at some common Big O classes and what they mean in terms of performance:
-
O(1) - Constant Time Complexity
-
Definition
The algorithm takes the same amount of time regardless of the input size.
-
Example
Accessing an element in an array by its index, which takes the same time regardless of array size.
1 2
def get_first_element(arr): return arr[0]
-
Explanation
The function above accesses the first element in a list. Regardless of how large the list grows, accessing the first element takes the same amount of time. Thus, it has a constant time complexity, O(1).
-
-
O(log n) - Logarithmic Time Complexity
-
Definition
In O(log n) complexity, the time required to run the algorithm increases logarithmically with the size of the input. This means that as the input size grows, the time taken increases slowly, in steps proportional to the logarithmic base (often base 2 for binary operations).
Typically, if the algorithm reduces the problem size by half with each step, the base is 2 (resulting in log₂(n) complexity), as seen in binary search.
However, if an algorithm divides the problem into k parts at each step, the base of the logarithm will be k i.e. O(logₖn). For example, if an algorithm splits the problem into three parts each time, the complexity would be O(log₃ n).
Logarithmic time complexity is highly efficient for large inputs because the time required grows very slowly, making these algorithms ideal for cases where the problem size can be repeatedly reduced by a constant factor.
-
Example
Performing a binary search on a sorted array, where the array is halved in each step.
1 2 3 4 5 6 7 8 9 10 11
def binary_search(arr, target): left, right = 0, len(arr) - 1 while left <= right: mid = (left + right) // 2 if arr[mid] == target: return mid elif arr[mid] < target: left = mid + 1 else: right = mid - 1 return -1
-
Explanation
The binary search algorithm divides the list in half on each iteration, reducing the search space exponentially. This results in a logarithmic time complexity, O(log₂n).
-
-
O(n) - Linear Time Complexity
-
Definition
The execution time grows proportionally with the input size.
-
Example
Finding the maximum element in an array by iterating through each item once.
1 2 3 4 5 6
def find_max(arr): max_value = arr[0] for num in arr: if num > max_value: max_value = num return max_value
-
Explanation
If you double the size of the array, the algorithm will take twice as long.
-
-
O(n log n) - Log-Linear Time Complexity
-
Definition
A combination of linear and logarithmic growth, typically seen in efficient sorting algorithms.
-
Example
Sorting an array using Merge Sort, where the array is split and merged recursively.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
def merge_sort(arr): if len(arr) <= 1: return arr mid = len(arr) // 2 left = merge_sort(arr[:mid]) right = merge_sort(arr[mid:]) return merge(left, right) def merge(left, right): result = [] while left and right: if left[0] < right[0]: result.append(left.pop(0)) else: result.append(right.pop(0)) result.extend(left or right) return result
-
Explanation
Sorting algorithms like merge sort break the input into smaller parts (log n times) and then recombine (n operations). Hence, O(n log n) complexity results from n operations done log n times.
-
-
O(n^2) - Quadratic Time Complexity
-
Definition
Execution time grows proportionally to the square of the input size, often due to nested loops.
-
Example
Sorting an array using Bubble Sort, where every element is compared with others in nested loops.
1 2 3 4 5 6 7
def bubble_sort(arr): n = len(arr) for i in range(n): for j in range(0, n-i-1): if arr[j] > arr[j+1]: arr[j], arr[j+1] = arr[j+1], arr[j] return arr
-
Explanation
Nested loops where each element is compared with every other element create this complexity, leading to quick growth with input size.
-
-
O(2^n) - Exponential Time Complexity
-
Definition
Execution time doubles with each additional input, making it impractical for large inputs.
-
Example
The
fibonacci(n)
function recursively calculates the nth Fibonacci number by summing the two preceding Fibonacci numbers, with the base case returningn
whenn
is 0 or 1.1 2 3 4
def fibonacci(n): if n <= 1: return n return fibonacci(n - 1) + fibonacci(n - 2)
-
Explanation
Recursive algorithms where the number of operations grows exponentially with n lead to this complexity. For fibonacci, each function call branches out into two more calls, doubling work at each step.
-
Comparing Different Time Complexities Through Visualization
To better understand Big O, let’s look at a hypothetical chart of how these different complexities grow as input size (n) increases.
Why Is Big O Important?
Big O Notation helps us:
- Predict Performance: By analyzing time complexity, we can predict how well an algorithm will perform as input size increases.
- Make Informed Choices: Understanding complexity allows us to choose algorithms that balance performance with resource constraints.
- Optimize Code: Big O encourages us to think about efficiency when coding, often leading to more efficient solutions.
Tips for Improving Algorithm Efficiency
- Choose Appropriate Data Structures: Selecting efficient data structures like dictionaries and sets can significantly improve performance.
- Minimize Nested Loops: Avoid unnecessary nested loops, as they often lead to O(n^2) complexity.
- Consider Recursive Alternatives: Recursive solutions can sometimes improve readability and performance for complex problems.
Conclusion
Big O Notation is essential for understanding and improving the efficiency of algorithms. By learning common Big O classes, we can better predict performance, make informed choices, and optimize our code.
In the next blog, we’ll delve into the essential mathematical concepts for Algorithmic Thinking, building a strong foundation to tackle complex algorithms with ease. See you there!